Maximize your JavaScript's efficiency by analyzing bottlenecks, minifying code, and caching smartly. Enhance your DOM manipulation with efficient handling and event techniques. Elevate algorithm performance with optimization strategies and Web Workers. Prioritize async operations for smoother tasks. Regular code audits maintain top-notch performance. If you want to unlock more ways to boost your JavaScript code, keep exploring the comprehensive guide.
Key Takeaways
- Analyze code for bottlenecks and hotspots
- Utilize profiling techniques for optimization
- Implement code minification and obfuscation
- Employ caching strategies for memory efficiency
- Prioritize lazy loading for faster initial load
Identifying Performance Bottlenecks
To pinpoint areas that slow down your code, analyze its execution for bottlenecks. When striving for efficient JavaScript code, utilizing profiling techniques is crucial. Profiling allows you to gather data on how your code runs, helping you identify hotspots that are potential bottlenecks.
Through hotspot analysis, you can focus on the specific areas that are causing your code to lag and optimize them effectively.
Profiling techniques involve tools that track the performance of your code, highlighting areas that require improvement. By examining the execution times of different functions and segments of your code, you can prioritize optimizing the parts that contribute most to the slowdown. Hotspot analysis zooms in on these critical sections, giving you a clear roadmap for enhancing your code's efficiency.
Minifying and Obfuscating Code
When it comes to optimizing your JavaScript code, minifying and obfuscating are crucial steps. By reducing the size of your code through minification and making it harder to understand through obfuscation, you can improve loading times and protect your code from being easily reverse-engineered.
These techniques play a significant role in enhancing the efficiency and security of your JavaScript applications.
Code Minification Benefits
Maximizing code efficiency involves leveraging the benefits of code minification, which entails compressing and obfuscating your code to optimize performance.
Code size reduction is a key advantage of minification, as it decreases the amount of data that needs to be transferred over the network, resulting in faster loading times for your web pages.
Additionally, resource optimization is achieved through minification, reducing the number of HTTP requests required to load a page. By minifying your code, unnecessary spaces, comments, and characters are removed, streamlining the codebase without affecting functionality.
This process not only enhances the speed and efficiency of your JavaScript code but also improves the overall user experience on your website.
Obfuscation Techniques Overview
By compressing and obfuscating your code, you can enhance its security and protect your intellectual property in addition to optimizing performance. When exploring code obfuscation techniques, keep in mind the security implications of obfuscation. Here are some key points to consider:
- Renaming Variables: Changes variable names to cryptic, non-descriptive terms.
- Removing Whitespace: Eliminates unnecessary spaces and line breaks to reduce file size.
- String Encryption: Encodes sensitive strings to make them harder to decipher.
- Control Flow Flattening: Restructures code to make it harder to follow the logic flow.
- Dead Code Injection: Introduces dummy code to confuse reverse engineers.
Embracing these techniques can fortify your code against prying eyes and enhance its overall protection.
Utilizing Caching Techniques
When optimizing your JavaScript code, utilizing caching techniques can greatly benefit you. Memory caching helps reduce time complexity, enhancing the overall performance of your applications.
Implementing smart data caching strategies can further improve the efficiency of your code.
Memory Caching Benefits
Consider incorporating memory caching techniques in your JavaScript code to enhance performance by reducing the need to repeatedly retrieve data from external sources. Memory caching offers various benefits, including:
- Cache expiration management: Implement strategies to handle cache expiration efficiently.
- Caching invalidation: Ensure that cached data is refreshed when it becomes outdated.
- Memory usage optimization: Utilize caching to reduce the amount of memory consumed by your application.
- Cache eviction strategies: Develop methods to decide which data to remove from the cache when it reaches its limit.
- Improved performance: By storing frequently accessed data in memory, you can significantly speed up your application. Take advantage of memory caching to boost your code's efficiency.
Time Complexity Reduction
To enhance the efficiency of your JavaScript code, leverage caching techniques to reduce time complexity. By implementing caching, you can store the results of expensive function calls and reuse them when needed, avoiding redundant computations. This approach significantly improves the performance of your code, especially in scenarios where the same calculations are repeated frequently.
When dealing with loops, consider loop optimization techniques to minimize the number of iterations and improve overall runtime. Additionally, conducting algorithm complexity analysis helps you identify bottlenecks and optimize critical sections of your code.
Data Caching Strategies
Implement caching strategies in your JavaScript code to optimize data retrieval and improve performance. When it comes to data storage optimization, cache invalidation is key. Consider both server-side caching and client-side caching strategies to enhance the efficiency of your code.
Here are five tips to help you make the most of caching techniques:
- Utilize server-side caching mechanisms for frequently accessed data.
- Implement client-side caching to store data locally and reduce server requests.
- Set expiration times for cached data to ensure it remains up to date.
- Use caching libraries like Redis or Memcached for efficient data storage.
- Regularly monitor and update your caching strategies to maintain optimal performance.
Implementing Lazy Loading Strategies
Boost your website's performance by incorporating efficient lazy loading strategies. Lazy loading images and deferred loading scripts are key techniques to optimize your website's loading speed. By implementing lazy loading for images, you can significantly reduce initial loading times, especially for pages with multiple images. This strategy ensures that images are only loaded when they're about to be viewed, rather than all at once when the page loads.
Deferred loading scripts work similarly by postponing the loading of scripts until they're actually needed, improving the initial loading speed of your website. This technique is particularly useful for large scripts that aren't crucial for the initial page render. By deferring their loading, you can prioritize essential content, making the user experience smoother and faster.
Incorporating lazy loading strategies not only enhances user experience but also boosts your website's performance, making it more efficient and responsive. By optimizing how resources are loaded, you can create a faster and more user-friendly website for your visitors.
Optimizing DOM Manipulation
Consider enhancing your website's performance by streamlining DOM manipulation for improved efficiency. To optimize DOM manipulation, focus on efficient event handling and streamlined data binding. Here are some tips to help you achieve this:
- Use Event Delegation: Delegate event handling to a common ancestor instead of binding events to multiple elements individually.
- Batch DOM Updates: Minimize the number of DOM manipulations by batching updates together.
- Leverage Virtual DOM: Consider using virtual DOM libraries to efficiently update the actual DOM based on changes.
- Avoid Inline Styles: Instead of directly applying styles to individual elements, utilize classes for styling and manipulate those classes.
- Cache DOM Queries: Store references to frequently accessed DOM elements to avoid redundant queries.
Leveraging Event Delegation
When handling multiple elements in your JavaScript code, leveraging event delegation can significantly improve performance effortlessly.
By targeting a common ancestor for these elements, you reduce the number of event listeners needed and streamline your code.
This approach not only optimizes efficiency but also enhances the overall user experience on your website.
Targeting Multiple Elements
To optimize your JavaScript code for efficiency, leverage event delegation when targeting multiple elements. This method allows you to attach a single event listener to a parent element, rather than individual listeners to each child element, enhancing element selection efficiency and enabling bulk element manipulation.
When implementing event delegation, follow these tips for a smoother coding experience:
- Reduce the number of event listeners attached to the document.
- Utilize event bubbling to capture events efficiently.
- Delegate events to the closest common ancestor of the target elements.
- Dynamically add or remove elements without worrying about event listeners.
- Improve overall performance by minimizing the impact of frequent DOM manipulations.
Improving Performance Effortlessly
Make your JavaScript code more efficient by mastering the art of event delegation, specifically when enhancing performance effortlessly.
By delegating event handling to a common ancestor rather than attaching event listeners to multiple elements, you can significantly reduce the number of event listeners in your code, leading to improved performance. This approach not only simplifies your code but also enhances browser compatibility and reduces the risk of errors.
Additionally, leveraging event delegation makes it easier to implement performance monitoring tools and conduct automated testing, ensuring that your code remains efficient and effective.
Embrace event delegation as a powerful strategy to streamline your JavaScript code and optimize its performance seamlessly.
Improving Algorithm Efficiency
Consider optimizing the algorithm efficiency through thoughtful design and analysis. Here are some key strategies to help you improve your code efficiency:
- Utilize Loop Optimization Techniques:
- Use iterators like `for` loops instead of `forEach`.
- Minimize the number of loops within loops for better performance.
- Implement Data Structure Enhancements:
- Choose the appropriate data structures like arrays or maps for faster access.
- Use Sets or Maps for unique data storage needs instead of arrays.
- Apply Memoization for Recurring Computations:
- Cache the results of expensive function calls to avoid redundant calculations.
- Avoid Unnecessary Operations:
- Refactor code to eliminate redundant or unnecessary operations.
- Opt for Binary Search over Linear Search:
- For large datasets, consider using binary search algorithms for quicker results.
Utilizing Web Workers for Multithreading
Enhance your JavaScript code efficiency by leveraging Web Workers for multithreading capabilities. By utilizing Web Workers, you can execute multiple scripts concurrently, improving the performance of your web applications. This approach allows tasks to run in the background without blocking the main thread, enabling smoother user experiences.
When implementing Web Workers, ensure cross-browser compatibility by checking for support in different browsers. While most modern browsers support Web Workers, it's essential to have fallback options for older versions. Additionally, consider error handling techniques to manage exceptions that may occur within the worker scripts. Implementing try-catch blocks and using the onerror event handler can help capture and handle errors effectively.
Prioritizing Asynchronous Operations
Prioritize asynchronous operations to boost the performance of your JavaScript code. When handling asynchronous tasks efficiently, you can significantly enhance the responsiveness and speed of your web applications. Here are some tips to help you optimize async functions and manage promises effectively:
- Use async/await: Simplify the handling of asynchronous operations by utilizing the async/await syntax.
- Avoid nested callbacks: Refactor your code to prevent callback hell and improve readability.
- Handle errors gracefully: Implement error handling mechanisms to prevent crashes and ensure smooth operation.
- Utilize Promise.all(): Improve performance by executing multiple promises concurrently using Promise.all().
- Consider using libraries: Explore libraries like Axios or Bluebird to enhance promise handling and streamline async operations.
Conducting Regular Code Audits
To ensure the efficiency and reliability of your JavaScript code, regularly conduct thorough code audits. Code review is essential for maintaining high-quality code that performs optimally. By reviewing your code regularly, you can identify and fix any issues, ensuring that your codebase remains efficient and scalable.
During these audits, focus on performance analysis as well. Evaluate the execution speed of your functions, the memory usage, and any potential bottlenecks that could impact the overall performance of your application. By conducting regular code audits, you can catch bugs early, optimize your code for better performance, and ensure that your JavaScript applications run smoothly.
Make code audits a part of your development process to maintain code quality and improve the overall user experience of your applications. Remember, a proactive approach to code maintenance through regular audits can save you time and effort in the long run.
Frequently Asked Questions
How Can I Optimize Memory Usage in Javascript Code?
To optimize memory usage in JavaScript code, focus on memory management techniques like efficient data structures and algorithms. Utilize garbage collection strategies to free up memory. Minify code and optimize resource utilization to enhance performance.
What Are the Best Practices for Handling Error Logging Efficiently?
When handling error logging efficiently, embrace error monitoring strategies to catch issues in real-time. Implement performance tuning techniques to minimize overhead. Ensure logs are concise yet informative. Regularly review and refine your logging process for optimal results.
Is There a Recommended Approach for Managing Dependencies in a Large Codebase?
When managing dependencies in a large codebase, consider strategies like using a package manager, version control, and modularization. Scalability considerations are crucial for maintaining an organized and efficient development environment.
How Can I Ensure Cross-Browser Compatibility While Maximizing Performance?
To ensure cross-browser compatibility while maximizing performance, focus on browser compatibility testing, performance optimization, JavaScript memory management, and efficient error handling. Implement these strategies for a smoother user experience across different browsers.
What Tools Can Help Automate Code Optimization Tasks Effectively?
To effectively automate code optimization tasks and enhance code quality, consider utilizing automation tools like Grunt or Gulp. These tools can help streamline your workflow, analyze performance metrics, and implement optimization techniques efficiently.
Conclusion
To maximize JavaScript code efficiency, it's crucial to identify performance bottlenecks. This involves minifying and obfuscating code, utilizing caching techniques, and implementing lazy loading strategies. Another key aspect is optimizing DOM manipulation and improving algorithm efficiency.
Additionally, utilizing web workers for multithreading, prioritizing asynchronous operations, and conducting regular code audits are essential steps. By following these strategies, you can ensure that your JavaScript code runs smoothly and efficiently, ultimately improving the overall performance of your web applications.
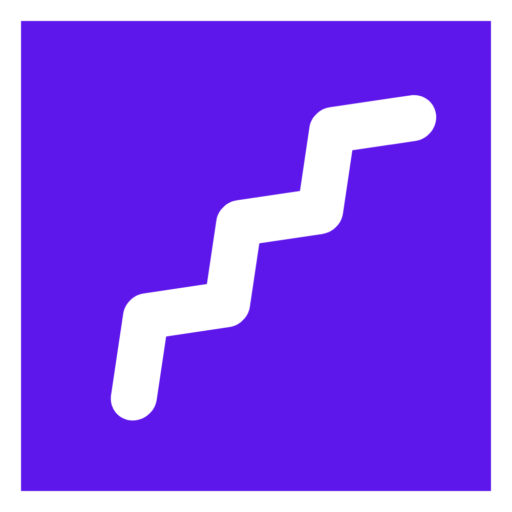
Pro Website Design San Jose brings over a decade of expertise in web development and SEO to Silicon Valley. Known for creating user-friendly, tailored websites, the Pro Website Design San Jose excels in meeting client-specific needs with innovative solutions, establishing itself as a trusted leader in the tech industry.