To enhance your web development, focus on improving JavaScript code performance. Identify bottlenecks using tools like Chrome DevTools to pinpoint slowdowns. Implement efficient data structures such as binary search trees and hash tables for optimized operations. Use caching techniques to store data and reduce recalculations. Minimize DOM manipulations and leverage asynchronous operations for better efficiency. These strategies will boost your project's performance significantly.
Key Takeaways
- Use code profiling tools to identify bottlenecks and optimize performance.
- Implement efficient data structures like binary search trees and hash tables.
- Utilize caching techniques to reduce repeated calculations and improve memory management.
- Minimize DOM manipulations by leveraging virtual DOM and event delegation.
- Leverage asynchronous operations for parallel processing and improved responsiveness.
Identifying Bottlenecks in Your Code
Identifying bottlenecks in your JavaScript code is crucial for optimizing its performance. Code profiling tools like Chrome DevTools can help you pinpoint areas of your code that are causing slowdowns. By analyzing the execution times of different functions and sections of your code, you can identify where optimizations are needed. Performance tuning involves making targeted changes to these bottleneck areas to improve overall efficiency.
When you dive into code profiling, focus on sections that are called frequently or involve complex operations. Look for loops, recursive functions, or operations that manipulate large amounts of data. These are common places where bottlenecks occur. Once you've identified these areas, consider ways to refactor your code for better performance. This could involve optimizing algorithms, reducing unnecessary calculations, or caching results to avoid repetitive computations.
Implementing Efficient Data Structures
To optimize the performance of your JavaScript code, consider implementing efficient data structures to enhance the overall efficiency of your program. Utilizing data structures such as binary search trees, hash tables, linked lists, and priority queues can significantly impact the speed and effectiveness of your code.
Here is a table showcasing these efficient data structures:
Data Structure | Description | Use Case |
---|---|---|
Binary Search Trees | Organizes data for efficient search operations | Searching for elements in a sorted collection |
Hash Tables | Stores key-value pairs for quick data retrieval | Implementing fast lookup tables for data management |
Linked Lists | A sequence of elements linked in a linear order | Managing dynamic data that requires frequent updates |
Priority Queues | Orders elements based on priority levels | Implementing algorithms like Dijkstra's shortest path |
Utilizing Caching Techniques
Consider leveraging caching techniques to optimize the performance of your JavaScript code. By utilizing caching, you can store frequently accessed data, such as API responses or computed values, to reduce the need for repeated calculations or network requests. This can significantly improve the speed of your application by cutting down on memory management overhead and mitigating network latency issues.
Caching helps in memory management by storing data in temporary storage, like the browser's cache or local storage, reducing the amount of memory your application needs to use for repetitive tasks. This can lead to faster load times and smoother user experiences, especially for data-intensive web applications.
Moreover, caching helps combat network latency by serving cached data locally instead of fetching it from a remote server every time. This minimizes the time it takes for data to travel across the network, improving the responsiveness of your application.
Incorporating caching techniques into your JavaScript code can thus enhance performance by optimizing memory usage and reducing the impact of network delays on your application's speed.
Minimizing DOM Manipulations
Minimize DOM manipulations to enhance JavaScript code performance. When you interact with the DOM frequently, it can lead to performance bottlenecks. One effective strategy to tackle this is by leveraging the concept of the Virtual DOM. The Virtual DOM is a lightweight copy of the actual DOM. When changes are made, they're first applied to the Virtual DOM, which then calculates the most efficient way to update the real DOM. This process reduces the number of direct manipulations on the actual DOM, resulting in improved performance.
Another technique to minimize DOM manipulations is through event delegation. Instead of attaching event handlers to multiple elements, you can utilize event delegation by adding a single event listener to a common parent element. This way, you can catch events as they bubble up the DOM tree. By handling events in this manner, you reduce the number of event listeners and enhance the performance of your JavaScript code.
Leveraging Asynchronous Operations
How can JavaScript code performance be enhanced through leveraging asynchronous operations?
By incorporating asynchronous operations, you can significantly boost the efficiency of your code. One key benefit is the ability to execute multiple tasks concurrently through parallel processing. This means that instead of waiting for one task to finish before starting the next, asynchronous operations allow tasks to run simultaneously, leading to faster overall execution.
Optimizing callbacks is another essential aspect of leveraging asynchronous operations. By utilizing callbacks effectively, you can ensure that functions are triggered only when certain conditions are met, preventing unnecessary delays in your code. This helps streamline the flow of your program and improves its performance by reducing idle time.
Incorporating asynchronous operations not only enhances the speed of your code but also contributes to a more responsive and user-friendly web application. By taking advantage of parallel processing and optimizing callbacks, you can unlock the full potential of JavaScript for web development.
Frequently Asked Questions
How Can I Optimize My Code for Mobile Devices?
To optimize your code for mobile devices, prioritize mobile responsiveness and user experience. Ensure cross-browser compatibility for better performance optimization. Keep your code lean and efficient to enhance the user experience across various devices.
What Tools Can Help Me Analyze Performance?
For analyzing performance, you should explore performance profiling tools. These tools offer real-time monitoring capabilities, helping you identify bottlenecks and optimize your code effectively. They provide insights crucial for enhancing your code's efficiency.
Should I Minify My Javascript Code for Better Performance?
Yes, you should minify your JavaScript code for better performance. It aids in reducing file sizes, improving load times, and enhancing browser caching. Additionally, consider implementing code splitting to optimize resource delivery for enhanced user experience.
Is There a Recommended Way to Handle Memory Leaks?
To handle memory leaks effectively, remember to utilize efficient garbage collection strategies and implement memory leak prevention techniques. Regularly audit your code, monitor memory usage, and clean up unused resources promptly for optimal performance.
How Can I Improve My Code's Accessibility and Seo?
To boost your code's accessibility and SEO, ensure cross-browser compatibility and use a semantic HTML structure. This approach allows for a more inclusive web experience, catering to a diverse audience and improving search engine rankings.
Conclusion
Overall, improving JavaScript code performance for web development is crucial for creating fast and efficient websites.
By identifying bottlenecks, implementing efficient data structures, utilizing caching techniques, minimizing DOM manipulations, and leveraging asynchronous operations, you can significantly enhance the speed and responsiveness of your web applications.
Remember to continuously analyze and optimize your code to ensure optimal performance for a seamless user experience.
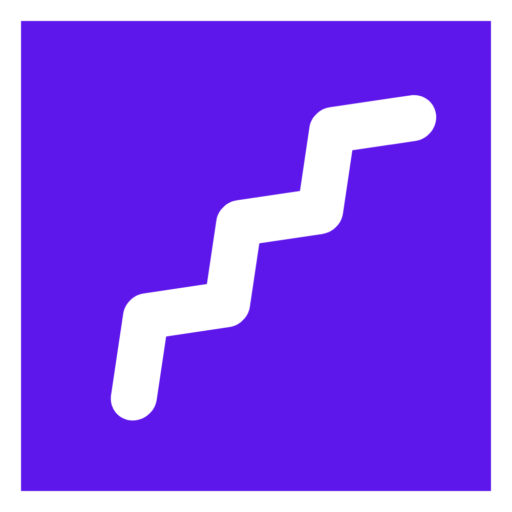
Pro Website Design San Jose brings over a decade of expertise in web development and SEO to Silicon Valley. Known for creating user-friendly, tailored websites, the Pro Website Design San Jose excels in meeting client-specific needs with innovative solutions, establishing itself as a trusted leader in the tech industry.