Master JavaScript event handling by grasping event propagation concepts. Visualize events triggering through the DOM tree for better understanding. Opt for event delegation to boost performance and simplify code maintenance. Avoid inline event handlers to enhance security and code organization. Keep HTML content clean for scalability and reusability. Ensure efficient event handling by limiting listeners and utilizing delegation wisely. Check cross-browser compatibility for robust performance. Follow security practices like input validation and avoiding risky functions. More in-depth tips await for maximizing your JavaScript event handling skills.
Key Takeaways
- Understand event propagation through the DOM tree.
- Implement event delegation for performance and maintenance benefits.
- Avoid inline event handlers for security and code organization.
- Utilize unobtrusive event handling for cleaner code.
- Ensure cross-browser compatibility for robust event handling.
Understand Event Propagation
To grasp event propagation effectively, focus on understanding the flow of events through the DOM hierarchy. Event propagation explanation is crucial for mastering JavaScript event handling. Imagine each element in the DOM as a node in a vast tree structure. When an event occurs on a particular element, it triggers a chain reaction that ripples through the DOM tree.
Event bubbling is a common form of event propagation where the event starts from the target element and bubbles up through its ancestors. Consider a scenario where you have nested elements like buttons inside a div. If you click the button, the click event will propagate not only to the button but also to the div containing it. Understanding these event bubbling case studies will help you anticipate how events will propagate through your document and handle them effectively at different levels of the DOM hierarchy.
Use Event Delegation Wisely
When considering event handling in JavaScript, it's important to utilize event delegation wisely to efficiently manage event propagation throughout the DOM hierarchy. Event delegation allows you to attach a single event listener to a parent element rather than to each individual child element, which enhances performance and simplifies code maintenance.
- Event delegation strategies:
- Identify a common ancestor element for the child elements you want to handle events on.
- Choose events that bubble up the DOM hierarchy to the specified ancestor.
- Use event.target to determine which specific child element triggered the event.
- Event delegation best practices:
- Avoid unnecessary event listeners on multiple elements.
- Dynamically add or remove child elements without worrying about event reattachment.
- Optimize performance by reducing the number of event listeners in the document.
Avoid Inline Event Handlers
When handling JavaScript events, it's crucial to steer clear of inline event handlers. They come with risks like decreased readability and maintenance challenges.
Instead, consider the benefits of event delegation for a more organized and efficient codebase.
Inline Handler Risks
Using inline event handlers in your JavaScript code can lead to potential security risks and maintenance challenges. When considering the freedom of your code, avoiding inline handlers is crucial. Here's why:
- Security Concerns: Inline event handlers mix HTML with JavaScript, making it easier for attackers to inject malicious code.
- Code Maintainability: Inline handlers scatter event handling logic throughout the HTML markup, making it harder to maintain and debug.
- Flexibility in Functionality: Separating event handling from HTML allows for more flexibility in reusing functions and modifying event behavior without changing the HTML structure.
Event Delegation Benefits
To enhance the security and maintainability of your code, consider leveraging the benefits of event delegation over using inline event handlers. Event delegation optimization allows you to attach a single event listener to a parent element rather than multiple listeners to individual child elements. This approach reduces memory consumption and improves performance, especially on large-scale projects with dynamic content.
By utilizing event delegation implementation strategies, such as utilizing event bubbling, you can streamline your code and make it more efficient. This method also simplifies adding or removing elements dynamically, as the parent element handles the events for its children.
Embracing event delegation not only enhances your code's organization but also contributes to a more robust and scalable JavaScript application.
Opt for Unobtrusive Event Handling
Consider incorporating event handlers into your HTML elements separate from the JavaScript logic for a cleaner and more maintainable codebase. This approach, known as unobtrusive event handling, allows you to keep your HTML content clean and focused on structure while enhancing the flexibility of your JavaScript code.
By separating the event handling from the markup, you create a more scalable and organized development environment.
- Separation of Concerns: Keeping event handling separate from the HTML promotes a clearer distinction between the structure of your page and the functionality it exhibits.
- Code Reusability: Unobtrusive event handling enables you to reuse event handlers across multiple elements without duplicating code.
- Enhanced Readability: By abstracting event handling to external scripts, your HTML becomes more readable, making it easier for developers to understand and modify the codebase.
Embracing unobtrusive event handling empowers you to build more robust and flexible web applications while maintaining a clean separation between structure and behavior.
Leverage Event Bubbling and Capturing
When handling events in JavaScript, understanding event bubbling and capturing is crucial. By leveraging event bubbling and capturing, you can efficiently manage event propagation.
Practical examples with code snippets will solidify your grasp on the differences between bubbling and capturing.
Event Flow Explanation
Understanding how event flow works in JavaScript can greatly enhance your ability to utilize event bubbling and capturing effectively in your web development projects.
When diving into event flow, consider the following:
- Event propagation clarification: Learn about how events move through the DOM tree.
- Event flow visualization: Visualize how events are processed from the target element to the top of the DOM.
- Understanding bubbling and capturing: Explore the differences between these two event propagation methods to leverage them in your projects effectively.
Bubbling Vs. Capturing
To effectively leverage event bubbling and capturing in your JavaScript projects, understanding the differences between these two event propagation methods is crucial. Event propagation nuances play a significant role in how events are handled in the DOM.
Bubbling starts from the target element and bubbles up through its ancestors, while capturing happens in the opposite direction, from the topmost ancestor down to the target element. When implementing event handling strategies, consider which phase – bubbling or capturing – best suits your needs.
Remember that most events bubble by default, but you can use event listeners with the `capture` option to switch to capturing mode. Experiment with both approaches to find the most efficient solution for your specific requirements.
Practical Examples With Code
Consider leveraging event bubbling and capturing to streamline your event handling process in JavaScript. By understanding event listener patterns, you can optimize your code for efficiency.
Here are some tips to enhance your event handling practices:
- Utilize event delegation: Instead of adding event listeners to multiple elements, attach a single event listener to a parent element.
- Take advantage of event bubbling: Allow events to propagate up the DOM tree, minimizing the number of event listeners needed.
- Use event capturing strategically: Capture events during the capturing phase to handle them before they reach the target element.
Implement Event Listener Efficiency
For better performance, ensure your event listeners are attached directly to the target elements. This approach, known as event listener optimization, enhances the efficiency of your code by reducing unnecessary event bubbling or capturing. By directly attaching event listeners to specific elements, you can streamline the event handling process, leading to a more responsive and faster user experience.
To further improve your event handling strategies, consider the following tips:
Efficient Event Handling Tips | Benefits |
---|---|
Attach listeners to target elements | Increases performance |
Use event delegation when possible | Optimizes memory usage |
Limit the number of event listeners | Reduces complexity |
Remove unnecessary listeners | Enhances readability |
Utilize event delegation wisely | Improves maintainability |
Handle Events Cross-Browser
When handling events across different web browsers, ensure consistent functionality and compatibility by addressing browser-specific event handling nuances.
To ensure seamless event handling across browsers, consider the following:
- Event Listener Compatibility: Make sure to use the appropriate methods for adding event listeners to elements. While `addEventListener` is widely supported, some older browsers might require fallbacks like `attachEvent` for compatibility.
- Browser Event Compatibility: Be mindful of differences in how browsers handle events. For instance, some browsers may have specific event properties or behaviors that need to be accounted for in your event handling code.
- Testing and Polyfills: Test your event handling code across various browsers to ensure it functions correctly. Consider using polyfills or libraries like Modernizr to handle inconsistencies and ensure compatibility with older browsers.
Practice Event Handling Security
Ensure event handling security by validating and sanitizing user input to prevent potential vulnerabilities. When implementing event handling best practices, always remember that user input can be manipulated to execute malicious code. By validating input data, you can ensure that only expected values are processed, reducing the risk of injection attacks.
Sanitizing user input by removing or escaping special characters further enhances event security measures, making it harder for attackers to exploit vulnerabilities in your code.
Another critical aspect of event handling security is to avoid using eval() or Function() constructors with user input, as these can execute arbitrary code and pose significant risks. Instead, rely on safer alternatives like JSON.parse) for parsing JSON data or specific validation functions tailored to your application's requirements.
Frequently Asked Questions
How Can I Prevent Event Propagation in Javascript?
To prevent event propagation in JavaScript, you can stop event bubbling by calling `event.stopPropagation()` or prevent event capturing with `event.stopImmediatePropagation()`. Use event listeners wisely and consider event delegation for efficient handling.
What Are the Drawbacks of Using Inline Event Handlers?
When using inline event handlers, you sacrifice code readability and risk performance impact. It's better to maintain clean code by separating your JavaScript from HTML. By doing this, you'll improve maintenance and scalability.
How Can I Ensure Cross-Browser Compatibility for Event Handling?
To ensure consistency across browsers for event handling, use a library like jQuery or a framework such as React. These tools manage cross-browser compatibility issues, allowing you the freedom to focus on building your project.
Why Is Event Delegation Considered a Best Practice?
Event delegation is a best practice due to its benefits of reducing the number of event listeners needed, improving performance by handling events at a higher level, and allowing for dynamic content handling.
What Security Risks Should I Be Aware of When Handling Events?
To prevent vulnerabilities, be cautious with dynamic event handlers, avoid inline event attributes, and sanitize user inputs to protect against XSS attacks. Follow event listener best practices to ensure secure and reliable event handling.
Conclusion
In conclusion, mastering JavaScript event handling is essential for creating interactive and efficient web applications. By understanding event propagation, using event delegation wisely, and implementing efficient event listeners, you can optimize your code and improve user experience.
Remember to handle events cross-browser, prioritize security, and avoid inline event handlers for a smoother development process. Keep practicing and experimenting with different event handling techniques to become a proficient JavaScript developer.
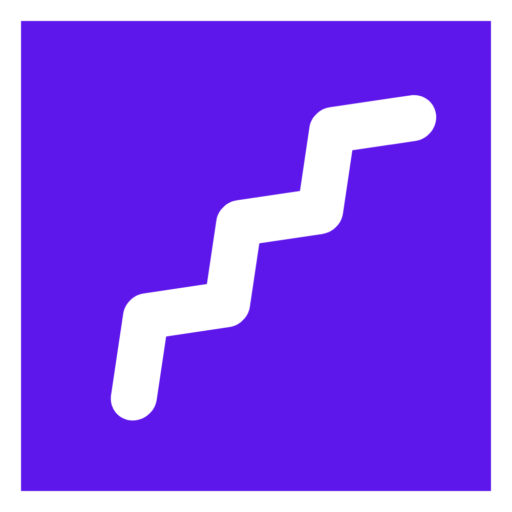
Pro Website Design San Jose brings over a decade of expertise in web development and SEO to Silicon Valley. Known for creating user-friendly, tailored websites, the Pro Website Design San Jose excels in meeting client-specific needs with innovative solutions, establishing itself as a trusted leader in the tech industry.