Explore cutting-edge front-end development with 7 advanced JavaScript techniques. Optimize DOM manipulation by batching changes and lazy loading resources. Dive into asynchronous programming using promise chaining and error handling. Master event handling for personalized interactions and efficient event delegation. Utilize design patterns like Singleton and Observer for scalable code. Enhance performance with Web Workers and virtual DOM efficiency. Leverage React reconciliation for faster rendering and component reusability. Implement Progressive Web Apps for offline functionality and improved user engagement. Elevate your web applications with these techniques and discover how they can transform your development process.
Key Takeaways
- Optimize DOM manipulation for performance gains.
- Implement asynchronous programming with promise chaining.
- Master event handling for efficient user interactions.
- Utilize design patterns for scalable code.
- Enhance performance with Web Workers for parallel processing.
Optimizing DOM Manipulation
When optimizing DOM manipulation, consider using efficient methods to improve performance. Batch processing allows you to group multiple operations together, reducing the number of times the DOM is accessed. This can significantly boost speed and responsiveness.
Instead of making individual changes one by one, you can bundle them and apply all at once. Lazy loading is another powerful technique. By loading only the necessary content initially and fetching additional resources as needed, you prevent unnecessary strain on the browser, leading to faster page loads and smoother user experiences.
Implementing Asynchronous Programming
To enhance your front-end development skills further, it's time to explore implementing asynchronous programming techniques. By utilizing promise chaining, you can manage multiple asynchronous operations effectively, ensuring smooth execution of tasks without blocking the main thread. This technique allows you to handle data fetching from APIs or databases seamlessly, enhancing the user experience on your website.
Integrating error handling in your asynchronous functions is crucial to gracefully manage any unexpected issues that may arise during data retrieval or processing. By implementing proper error-catching mechanisms, you can prevent your application from crashing and provide users with informative error messages when something goes wrong.
Moreover, the async/await syntax offers a cleaner and more readable way to work with asynchronous code, making your development process more efficient. This modern approach simplifies the handling of promises, allowing you to write asynchronous functions that resemble synchronous ones, improving code maintainability and readability. Embrace these advanced techniques to elevate your front-end development skills and create robust, high-performing web applications.
Mastering Event Handling
Mastering event handling in JavaScript involves efficiently managing user interactions and system events to create dynamic and responsive web applications. To enhance your understanding, let's delve into some key concepts in event handling:
Concept | Description |
---|---|
Custom Event Listeners | Create personalized event handlers tailored to your specific application requirements. |
Event Delegation | Delegate event handling to a common parent element, reducing the number of event listeners. |
Event Bubbling | Events triggered on nested elements will "bubble up" through their ancestors in the DOM tree. |
Understanding event bubbling helps you optimize event handling by leveraging the bubbling phase to capture events at higher levels in the DOM. Custom event listeners empower you to tailor interactions precisely to your needs, enhancing user experiences. Implementing event delegation efficiently manages event listeners, maintaining a responsive web application. By mastering these techniques, you gain the freedom to create dynamic and interactive web solutions that engage users effectively.
Utilizing Design Patterns
Utilizing design patterns in front-end development can greatly enhance the structure and scalability of your codebase. By implementing patterns like the Singleton pattern, you ensure that only one instance of a class is created, which can be beneficial for managing global state or resources efficiently.
The Factory pattern, on the other hand, provides a way to create objects without specifying the exact class of object that will be created, allowing for more flexible and decoupled code.
When it comes to managing complex interactions and dependencies between objects, the Observer pattern shines. It establishes a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. This is particularly useful in scenarios where multiple parts of your application need to react to changes in a consistent manner.
Additionally, the Strategy pattern allows you to define a family of algorithms, encapsulate each one, and make them interchangeable. This promotes code reusability and enables you to switch between different algorithms dynamically at runtime.
Embracing these design patterns empowers you to write more modular, maintainable, and extensible front-end code.
Enhancing Performance With Web Workers
Improving performance in front-end development can be achieved through the use of Web Workers. Web worker communication allows you to run scripts in the background, separate from the main thread, enabling tasks to be processed without affecting the user interface responsiveness. By utilizing Web Workers for parallel processing, you can enhance your application's speed and efficiency.
One of the key benefits of Web Workers is the ability to perform tasks concurrently, taking advantage of multi-core processors. This parallel processing capability enables complex operations to be divided into smaller sub-tasks that can be executed simultaneously, resulting in faster overall execution times.
Moreover, Web Workers facilitate efficient communication between different parts of your application, enabling seamless data exchange without blocking the main thread. This asynchronous communication mechanism ensures that your front-end remains smooth and responsive, even when handling resource-intensive operations. By harnessing the power of Web Workers, you can significantly boost the performance of your web applications and deliver a seamless user experience.
Utilizing Virtual DOM for Efficiency
When working on front-end development projects, you should understand the basics of Virtual DOM to enhance your efficiency.
Implementing performance optimization tips can significantly improve the speed and responsiveness of your web applications.
It's crucial to grasp the React reconciliation process to efficiently manage updates and changes in your Virtual DOM.
Virtual DOM Basics
Implementing Virtual DOM in your front-end development process can significantly enhance the efficiency of your web applications. The Virtual DOM benefits include faster rendering, improved performance, and a smoother user experience.
By utilizing Virtual DOM, you can minimize the number of direct DOM manipulations, leading to better optimization and reduced rendering time. However, Virtual DOM does come with some drawbacks, such as increased memory usage and the initial setup cost.
To implement Virtual DOM effectively, consider using libraries like React or Vue.js, which handle the Virtual DOM updates efficiently. By employing key strategies like batch updates and smart diffing algorithms, you can make the most of Virtual DOM and elevate the performance of your front-end applications.
Performance Optimization Tips
To boost the efficiency of your front-end development, leverage Virtual DOM for enhanced performance optimization. By utilizing Virtual DOM, changes are first made to a virtual representation of the actual DOM, allowing for quicker updates and reducing the need for direct manipulation of the real DOM.
Alongside this, consider implementing browser caching strategies to store frequently accessed resources locally, reducing load times and improving overall performance.
Additionally, employing code splitting techniques can help in breaking down your codebase into smaller chunks, enabling selective loading of only necessary components, thus optimizing the loading speed of your web application.
These strategies, when combined with Virtual DOM utilization, can significantly enhance the performance of your front-end projects.
React Reconciliation Process
Leveraging the React reconciliation process through Virtual DOM can significantly enhance the efficiency of your front-end development projects. By utilizing Virtual DOM, React minimizes unnecessary re-renders, optimizing performance.
This process is crucial for efficient state management, ensuring that only components affected by state changes are updated. Additionally, Virtual DOM allows for enhanced component reusability, as changes are efficiently reconciled and rendered only where needed.
This approach streamlines the updating process, resulting in faster and more responsive user interfaces. Embracing the React reconciliation process not only improves the performance of your application but also simplifies state management and promotes the reuse of components throughout your projects.
Implementing Progressive Web Apps
By incorporating service workers and caching strategies, you can enhance user experience in your web applications while implementing Progressive Web Apps. Progressive Web Apps (PWAs) offer offline functionality and push notifications, making them a powerful tool for engaging users even when they are not actively using your site. Service workers enable offline functionality by allowing your web app to cache important assets, ensuring that users can still access content even without an internet connection. Push notifications, on the other hand, help you re-engage users by sending them timely updates and alerts.
To emphasize the benefits of implementing Progressive Web Apps, consider the following table:
Feature | Description | Benefits |
---|---|---|
Offline Functionality | Enable users to access content offline | Increased user engagement |
Push Notifications | Send timely updates to users | Higher user retention |
Improved Performance | Faster loading times | Enhanced user experience |
Frequently Asked Questions
How Can I Handle Memory Leaks Caused by DOM Manipulation?
When handling memory leaks caused by DOM manipulation, focus on garbage collection optimization. Monitor performance and profile your code to pinpoint areas causing issues. This approach will help you efficiently manage memory and improve overall performance.
What Are the Best Practices for Error Handling in Asynchronous Programming?
When handling errors in async code, use timeout strategies to prevent hanging tasks. Avoid callback hell by leveraging promise chaining for cleaner code. Ensure proper error propagation to handle exceptions effectively and maintain code readability.
How Can I Prioritize and Manage Event Handlers Efficiently?
To prioritize and manage event handlers efficiently, focus on event delegation for better performance optimization. Avoid callback hell by organizing code logically. Remember to keep an eye on garbage collection to maintain a lean application.
Are There Any Anti-Patterns to Avoid When Using Design Patterns?
When using design patterns, steer clear of common mistakes like overcomplicating solutions or blindly following patterns without understanding the problem. Look out for code smells that might indicate design pattern pitfalls. Stay vigilant!
How Can I Optimize Communication Between Web Workers and the Main Thread?
To optimize communication between web workers and the main thread, ensure message passing efficiency. Employ thread synchronization techniques for smooth interaction. Keep interactions concise and synchronized to enhance overall performance and responsiveness of your web application.
Conclusion
You've learned some advanced JavaScript techniques for front-end development that can take your skills to the next level. By optimizing DOM manipulation, mastering event handling, and implementing design patterns, you can create more efficient and responsive web applications.
Utilizing asynchronous programming and web workers can enhance performance, while virtual DOM can improve efficiency. By implementing progressive web apps, you can stay ahead of the curve in the ever-evolving world of front-end development.
Keep practicing and experimenting to become a master front-end developer.
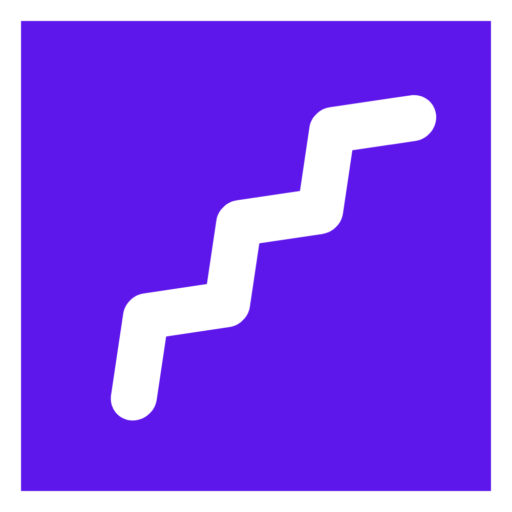
Pro Website Design San Jose brings over a decade of expertise in web development and SEO to Silicon Valley. Known for creating user-friendly, tailored websites, the Pro Website Design San Jose excels in meeting client-specific needs with innovative solutions, establishing itself as a trusted leader in the tech industry.